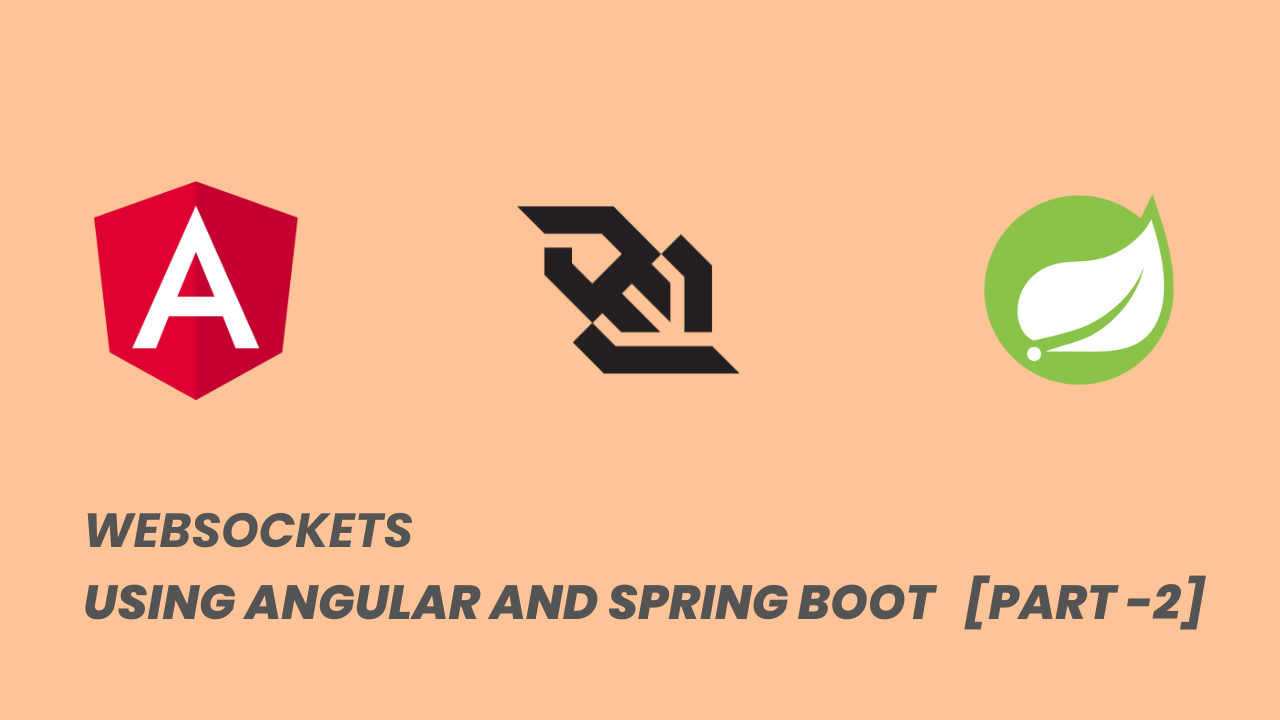
Building a Real-Time WebSocket Client with Angular [Part-2]
In the previous article, we set up a WebSocket server using Spring Boot. Now, let’s move to the client side and integrate this WebSocket server with an Angular application to receive real-time updates. This article will guide you through creating an Angular WebSocket client that subscribes to the events sent by the Spring Boot server.
Table Of Contents
- 1.Introduction
- 2.Setting Up the Angular Project
- 3.Adding WebSocket Support in Angular
- 4.Implementing the WebSocket Client Service
- 5.Displaying Real-Time Updates
- 6.Testing the Application
- 7.Conclusion
Introduction
In this part, we’ll set up an Angular app to connect to our Spring Boot WebSocket server, listening for real-time events and dynamically displaying these updates. This type of setup is useful for live feeds, notifications, and any application where users benefit from immediate updates.
Setting Up the Angular Project
Step 1: Create a new Angular project
ng new websocket-angular-client
cd websocket-angular-client
Step 2: Install the WebSocket dependency:
npm install rxjs
3. Adding WebSocket Support in Angular
In Angular, we’ll use RxJS's WebSocketSubject for WebSocket connections. This makes handling real-time data streams easier with RxJS operators like subscribe, map, and filter.
Step 1: Create a WebSocketService to handle WebSocket communication:
ng generate service services/WebSocket
Step 2: Open the generated web-socket.service.ts file and set up the connection.
4. Implementing the WebSocket Client Service
In this service, we’ll connect to the WebSocket server and subscribe to its messages.
// src/app/services/web-socket.service.ts
import { Injectable } from '@angular/core';
import { WebSocketSubject } from 'rxjs/webSocket';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root',
})
export class WebSocketService {
private socket$: WebSocketSubject;
constructor() {
this.connect();
}
private connect(): void {
this.socket$ = new WebSocketSubject('ws://localhost:8080/ws/events');
// Log messages received from the server
this.socket$.subscribe(
message => console.log('Message received: ', message),
err => console.error('Error: ', err),
() => console.warn('WebSocket connection closed')
);
}
public sendMessage(message: any): void {
this.socket$.next(message);
}
public getMessages(): Observable {
return this.socket$.asObservable();
}
}
In this service:
- connect() initializes the WebSocket connection to the server at ws://localhost:8080/ws/events.
- sendMessage() sends messages to the server.
- getMessages() exposes an observable for the incoming messages.
5. Displaying Real-Time Updates
To make use of the WebSocket service, let’s create a component to display the real-time updates.
Step 1: Generate a new component to display events:
ng generate component components/EventViewer
Step 2: In event-viewer.component.ts, use the WebSocket service to subscribe to real-time messages and display them..
// src/app/components/event-viewer/event-viewer.component.ts
import { Component, OnInit } from '@angular/core';
import { WebSocketService } from '../../services/web-socket.service';
@Component({
selector: 'app-event-viewer',
templateUrl: './event-viewer.component.html',
styleUrls: ['./event-viewer.component.css']
})
export class EventViewerComponent implements OnInit {
events: string[] = [];
constructor(private webSocketService: WebSocketService) {}
ngOnInit(): void {
this.webSocketService.getMessages().subscribe(message => {
this.events.push(message);
});
}
}
Step 3: Create a basic HTML template in event-viewer.component.html to display the events:
<div class="event-viewer">
<h3>Real-Time Events</h3>
<ul>
<li *ngFor="let event of events">{{ event }}</li>
</ul>
</div>
Step 4: Add the EventViewerComponent to your main application template, typically in app.component.html:
<app-event-viewer></app-event-viewer>
6. Testing the Application
- 1.Run the Spring Boot server to ensure WebSocket events are being generated.
- 2.Start the Angular application by running:.
- 3.Open http://localhost:4200 in your browser to see the real-time updates displayed on the page.
ng serve
Each new event from the server should immediately appear in the event list, demonstrating successful WebSocket communication between the Angular client and the Spring Boot WebSocket server.
7. Conclusion
In this article, we demonstrated how to integrate an Angular client with a Spring Boot WebSocket server for real-time communication. This approach enables seamless, live updates across the application, useful for any dynamic data-driven app.
In future articles, we’ll explore additional features, such as implementing custom messaging and managing connection states for a more robust WebSocket client.